public class TagDispatch extends Activity {
private TextView mTextView;
private NfcAdapter mNfcAdapter;
private PendingIntent mPendingIntent;
private IntentFilter[] mIntentFilters;
private String[][] mNFCTechLists;
ImageView getncView;
String ncimage;
@Override
public void onCreate(Bundle savedState) {
super.onCreate(savedState);
setContentView(R.layout.main_nfc);
mTextView = (TextView)findViewById(R.id.tv1);
getncView = (ImageView) findViewById(R.id.getncView);
mNfcAdapter = NfcAdapter.getDefaultAdapter(this);
if (mNfcAdapter != null) {
mTextView.setText("상대방 폰과 가까이 대주세요");
} else {
mTextView.setText("이 폰은 NFC 기능이 없습니다.");
}
// create an intent with tag data and deliver to this activity
mPendingIntent = PendingIntent.getActivity(this, 0,
new Intent(this, getClass()).addFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP), 0);
// set an intent filter for all MIME data
IntentFilter ndefIntent = new IntentFilter(NfcAdapter.ACTION_NDEF_DISCOVERED);
try {
ndefIntent.addDataType("*/*");
mIntentFilters = new IntentFilter[] { ndefIntent };
} catch (Exception e) {
Log.e("TagDispatch", e.toString());
}
mNFCTechLists = new String[][] { new String[] { NfcF.class.getName() } };
}
@Override
public void onNewIntent(Intent intent) {
String action = intent.getAction();
Tag tag = intent.getParcelableExtra(NfcAdapter.EXTRA_TAG);
String s = action + "\n\n" + tag;
// parse through all NDEF messages and their records and pick text type only
Parcelable[] data = intent.getParcelableArrayExtra(NfcAdapter.EXTRA_NDEF_MESSAGES);
if (data != null) {
try {
for (int i = 0; i < data.length; i++) {
NdefRecord[] recs = ((NdefMessage)data[i]).getRecords();
for (int j = 0; j < recs.length; j++) {
if (recs[j].getTnf() == NdefRecord.TNF_WELL_KNOWN &&
Arrays.equals(recs[j].getType(), NdefRecord.RTD_TEXT)) {
byte[] payload = recs[j].getPayload();
String textEncoding = ((payload[0] & 0200) == 0) ? "UTF-8" : "UTF-16";
int langCodeLen = payload[0] & 0077;
s += ("\n\nNdefMessage[" + i + "], NdefRecord[" + j + "]:\n\"" +
new String(payload, langCodeLen + 1, payload.length - langCodeLen - 1, textEncoding) +
"\"");
// [B@34e6cba2
if(j == 7) {
ncimage = (new String(payload, langCodeLen + 1, payload.length - langCodeLen - 1, textEncoding));
byte[] buffers = ncimage.getBytes();
getncView.setImageBitmap(BitmapFactory.decodeByteArray(buffers, 0, buffers.length));
}
}
}
}
} catch (Exception e) {
Log.e("TagDispatch", e.toString());
}
}
mTextView.setText(s);
}
@Override
public void onResume() {
super.onResume();
if (mNfcAdapter != null)
mNfcAdapter.enableForegroundDispatch(this, mPendingIntent, mIntentFilters, mNFCTechLists);
}
@Override
public void onPause() {
super.onPause();
if (mNfcAdapter != null)
mNfcAdapter.disableForegroundDispatch(this);
}
}
위 소스가 NFC를 받는 java 파일이구요.
public class BeamData extends Activity {
@Override
public void onCreate(Bundle savedState) {
super.onCreate(savedState);
setContentView(R.layout.nfc);
mPreviewImage = (ImageView) findViewById(R.id.selImageView);
Intent intent = getIntent();
code = intent.getStringExtra("code");
name = intent.getStringExtra("name");
email = intent.getStringExtra("email");
phone = intent.getStringExtra("phone");
cname = intent.getStringExtra("cname");
caddr = intent.getStringExtra("caddr");
cposition = intent.getStringExtra("cposition");
curl = intent.getStringExtra("curl");
name_nfc = (TextView) findViewById(R.id.name_nfc);
email_nfc = (TextView) findViewById(R.id.email_nfc);
phone_nfc = (TextView) findViewById(R.id.phone_nfc);
cname_nfc = (TextView) findViewById(R.id.cname_nfc);
caddr_nfc = (TextView) findViewById(R.id.caddr_nfc);
cposition_nfc = (TextView) findViewById(R.id.cposition_nfc);
curl_nfc = (TextView) findViewById(R.id.curl_nfc);
name_nfc.setText(name);
email_nfc.setText(email);
phone_nfc.setText(phone);
cname_nfc.setText(cname);
caddr_nfc.setText(caddr);
cposition_nfc.setText(cposition);
curl_nfc.setText(curl);
showImg_nfc();
mTextView = (TextView)findViewById(R.id.tv);
mNfcAdapter = NfcAdapter.getDefaultAdapter(this);
if (mNfcAdapter != null) {
mTextView.setText("상대방 폰을 가까이 가져가 주세요.");
} else {
mTextView.setText("This phone is not NFC enabled.");
}
// create an NDEF message with two records of plain text type
mNdefMessage = new NdefMessage(
new NdefRecord[] {
createNewTextRecord(name, Locale.KOREAN, true),
createNewTextRecord(email, Locale.ENGLISH, true),
createNewTextRecord(phone, Locale.ENGLISH, true),
createNewTextRecord(cname, Locale.KOREAN, true),
createNewTextRecord(caddr, Locale.KOREAN, true),
createNewTextRecord(cposition, Locale.KOREAN, true),
createNewTextRecord(curl, Locale.ENGLISH, true),
createNewTextRecord(buffersArrayString, Locale.ENGLISH, true)
});
}
public static NdefRecord createNewTextRecord(String text, Locale locale, boolean encodeInUtf8) {
byte[] langBytes = locale.getLanguage().getBytes(Charset.forName("US-ASCII"));
Charset utfEncoding = encodeInUtf8 ? Charset.forName("UTF-8") : Charset.forName("UTF-16");
byte[] textBytes = text.getBytes(utfEncoding);
int utfBit = encodeInUtf8 ? 0 : (1 << 7);
char status = (char)(utfBit + langBytes.length);
byte[] data = new byte[1 + langBytes.length + textBytes.length];
data[0] = (byte)status;
System.arraycopy(langBytes, 0, data, 1, langBytes.length);
System.arraycopy(textBytes, 0, data, 1 + langBytes.length, textBytes.length);
return new NdefRecord(NdefRecord.TNF_WELL_KNOWN, NdefRecord.RTD_TEXT, new byte[0], data);
}
@Override
public void onResume() {
super.onResume();
if (mNfcAdapter != null)
mNfcAdapter.enableForegroundNdefPush(this, mNdefMessage);
}
@Override
public void onPause() {
super.onPause();
if (mNfcAdapter != null)
mNfcAdapter.disableForegroundNdefPush(this);
}
private void showImg_nfc() {
dbHandler = new DBHandler(this);
try{
// String sql1 = "select ncimg from members where "+ code;
// Cursor cursor = db.rawQuery("select code from members", null);
cursor = dbHandler.selectNC();
cursor.moveToLast();
byte[] image = cursor.getBlob(cursor.getColumnIndex("ncimg"));
// Bitmap bm = BitmapFactory.decodeByteArray(image, 0, image.length);
buffersArrayString = image.toString();
bitmap = BitmapFactory.decodeByteArray(image, 0, image.length);
mPreviewImage.setImageBitmap(bitmap);
cursor.close();
}catch (Exception e){
e.printStackTrace();
}
}
}
위가 NFC로 데이터를 보내는 java입니다.
showImg_nfc에서 DB에 있는 이미지를 byte로 불러온후 NFC로 전송하기 위해서 buffersArrayString 에 String으로 저장한뒤 NFC로 전송하게 했습니다.
그리고 받는 데에선 7번째로 받은 값을 ncimg에 저장하고 ncimg를 byte로 변환한뒤 getncView라는 ImageView에 보여주려고 하는데요.
이렇게 서로 데이터를 교환하면
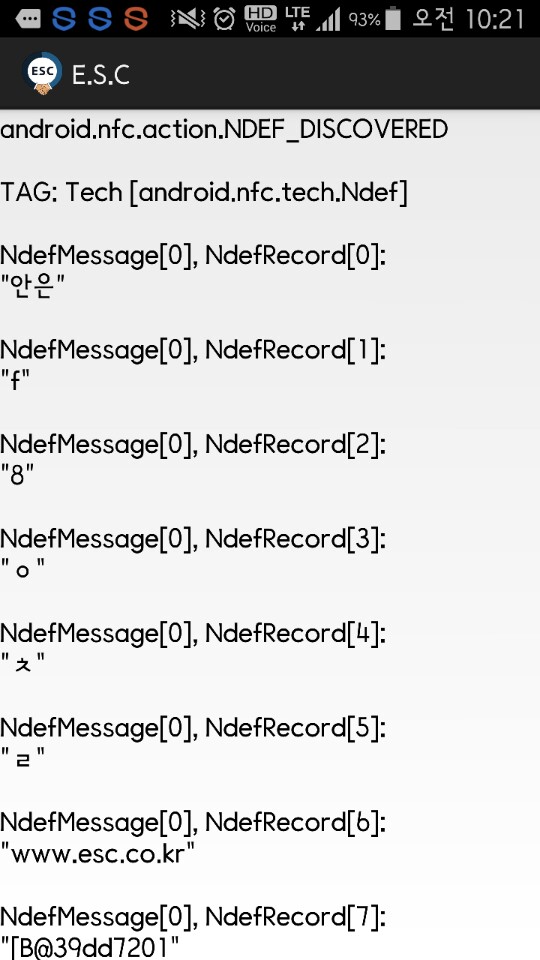
왼쪽이 보내는 화면이고 오른쪽이 받은 화면입니다.
맨 마지막 7번째에서 이미지를 String으로 받아 온 것까지는 된 것 같습니다.
그런데 이미지가 안보이네요....
이게 오류 로그인데.. (오류로그는 댓글로)
저걸 대충보면 12byte가 필요한데 10byte밖에 없다는 뜻.. ? 인걸로 보입니다.
근데 String으로 받았던 값을 보면 정확히 다 들어 온 것으로 보이는데 ... 왜 이런 현상이 일어나는지 모르겠네요...
안드로이드 초보라서 NFC기능이 너무 어렵습니다... 도와주세요 개발자님들 ㅠㅠ