암호화 알고리즘을 안드로이드 디바이스에서 시간 측정 테스트를 하는데요
왜 할때마다 계속 데이터가 일정하지 않고 차이가 많이 나는 걸까요 ?
제가 혹시 소스를 잘못 한건지 해서 제가 테스트에 사용한 소스와 테스트 결과를 첨부합니다.
아시는 분들은 답변좀 해주세요. 위에 분이 테스트 결과 보고
왜 똑같은 데이터로 암복호화를 하는데 시간 차이가 이렇게 많이 나냐고 해서요
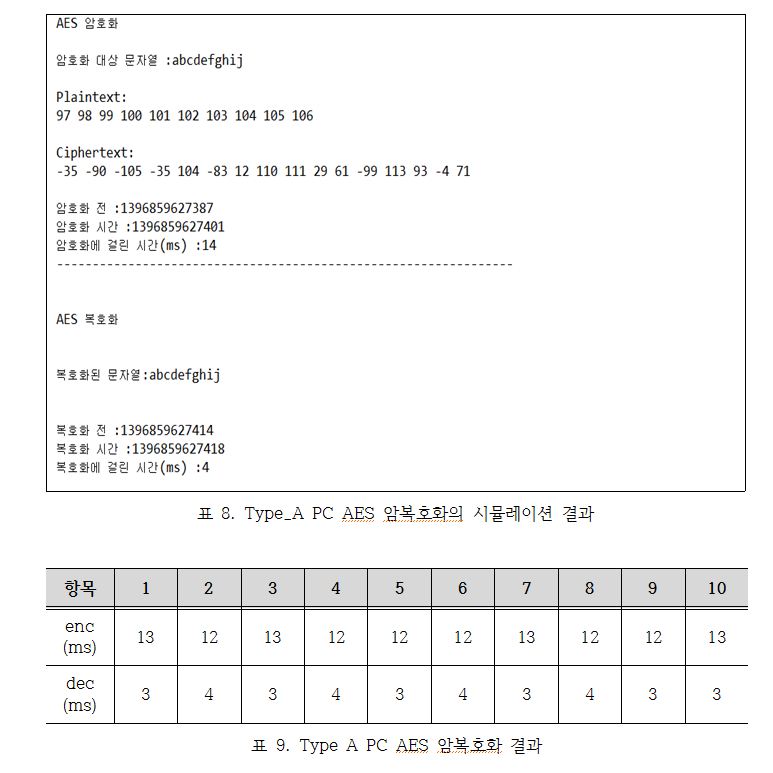
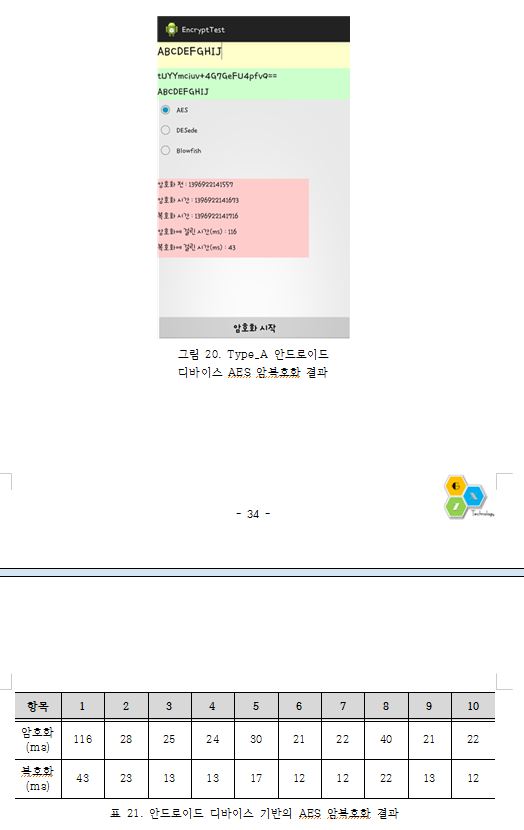
package com.exam.encrypttest;
import java.security.NoSuchAlgorithmException;
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.TextView;
public class MainActivity extends Activity {
TextView tv1, tv2, tv3, tv4, tv5, tv6, tv7;
EditText edit1;
Button but1;
RadioButton radio1, radio2, radio3;
String sendtext;
byte[] decrypted;
byte[] encrypted;
String type = "AES";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tv1 = (TextView) findViewById(R.id.text01);
tv2 = (TextView) findViewById(R.id.text02);
tv3 = (TextView) findViewById(R.id.text03);
tv4 = (TextView) findViewById(R.id.text04);
tv5 = (TextView) findViewById(R.id.text05);
tv6 = (TextView) findViewById(R.id.text06);
tv7 = (TextView) findViewById(R.id.text07);
edit1 = (EditText) findViewById(R.id.edit01);
but1 = (Button) findViewById(R.id.button01);
radio1 = (RadioButton) findViewById(R.id.radio01);
radio2 = (RadioButton) findViewById(R.id.radio02);
radio3 = (RadioButton) findViewById(R.id.radio03);
edit1.setOnClickListener(new ButtonClick());
;
radio1.setOnClickListener(new ButtonClick());
radio2.setOnClickListener(new ButtonClick());
radio3.setOnClickListener(new ButtonClick());
but1.setOnClickListener(new ButtonClick());
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
class ButtonClick implements View.OnClickListener {
public void onClick(View v) {
int sel = v.getId();
switch (sel) {
case R.id.button01:
Encryption(edit1.getText().toString(), type);
break;
case R.id.radio01:
tv1.setText("");
tv2.setText("");
tv3.setText("");
tv4.setText("");
tv5.setText("");
tv6.setText("");
tv7.setText("");
type = radio1.getText().toString();
break;
case R.id.radio02:
tv1.setText("");
tv2.setText("");
tv3.setText("");
tv4.setText("");
tv5.setText("");
tv6.setText("");
tv7.setText("");
type = radio2.getText().toString();
break;
case R.id.radio03:
tv1.setText("");
tv2.setText("");
tv3.setText("");
tv4.setText("");
tv5.setText("");
tv6.setText("");
tv7.setText("");
type = radio3.getText().toString();
break;
}
}
private void Encryption(String text, String key) {
Cipher cipher;
SecretKeySpec skeySpec;
KeyGenerator kgen;
try {
kgen = KeyGenerator.getInstance(key);
SecretKey skey = kgen.generateKey();
if(key.equals("DESede"))
{
kgen.init(168);
}
else
{
kgen.init(128);
}
byte[] raw = skey.getEncoded(); // 키값 생성
skeySpec = new SecretKeySpec(raw, key);
cipher = Cipher.getInstance(key);
long time1 = System.currentTimeMillis();
tv3.setText("암호화 전 : "+time1);
byte[] encrypted = Encrypt(text, skeySpec, cipher);
sendtext = Base64.encode(encrypted);
tv1.setText(sendtext);
long time2 = System.currentTimeMillis();
tv4.setText("암호화 시간 : "+time2);
byte[] abc = Base64.decode(sendtext);
decrypted = Decrypt(abc, skeySpec, cipher);
tv2.setText(new String(decrypted));
long time3 = System.currentTimeMillis();
tv5.setText("복호화 시간 : "+time3);
tv6.setText("암호화에 걸린 시간(ms) : "+(time2-time1));
tv7.setText("복호화에 걸린 시간(ms) : "+(time3-time2));
} catch (NoSuchAlgorithmException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (NoSuchPaddingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ArrayIndexOutOfBoundsException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public byte[] Encrypt(String data, SecretKeySpec keySpec,
Cipher cipher) throws Exception, ArrayIndexOutOfBoundsException {
// 암호화
cipher.init(Cipher.ENCRYPT_MODE, keySpec); // 암호화 모드로 지정
for(int i=0;i<=8000;i++){
encrypted = cipher.doFinal(data.getBytes());
}
return encrypted;
}
public byte[] Decrypt(byte[] encrypted_data,
SecretKeySpec keySpec, Cipher cipher) throws Exception,
ArrayIndexOutOfBoundsException {
// 복호화
cipher.init(Cipher.DECRYPT_MODE, keySpec); // 복호화 모드로 지정
for(int i=0;i<=8000;i++){
decrypted = cipher.doFinal(encrypted_data);
}
return decrypted;
}
}
}