package com.example.sangjun.nulsecall;
import android.content.Intent;
import android.graphics.Bitmap;
import android.os.AsyncTask;
import android.os.Handler;
import android.os.StrictMode;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class login extends AppCompatActivity {
Button p_regist;
Button n_regist;
Button p_page;
Button n_page;
EditText id;
EditText password;
String userId;
String userPw;
Handler mHandler;
public GettingPHP gPHP;
TextView tv;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
p_regist = (Button)findViewById(R.id.button2);
n_regist = (Button)findViewById(R.id.button3);
p_page = (Button)findViewById(R.id.button4);
n_page = (Button)findViewById(R.id.button7);
id = (EditText)findViewById(R.id.editText);
password = (EditText)findViewById(R.id.editText2);
tv = (TextView)findViewById(R.id.textView22);
p_regist.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent p_regist_intent= new Intent(login.this, p_regist.class);
startActivity(p_regist_intent);
}
});
n_regist.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent n_regist_intent= new Intent(login.this, n_regist.class);
startActivity(n_regist_intent);
}
});
p_page.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Intent p_page_intent= new Intent(login.this, p_page.class);
//startActivity(p_page_intent);
// new ReadJSONFeed().execute("http://165.194.34.207:80/loginInfo.php");
String inputId=id.getText().toString();
String inputPass= password.getText().toString();
String url= "http://165.194.34.207:80/loginInfo.php";
// loginMysql(inputId,inputPass,url);
gPHP = new GettingPHP();
gPHP.execute(url);
}
});
n_page.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent n_page_intent = new Intent(login.this, n_page.class);
startActivity(n_page_intent);
}
});
}
class GettingPHP extends AsyncTask<String, Integer, String> {
@Override
protected String doInBackground(String... params) {
StringBuilder jsonHtml = new StringBuilder();
try {
URL phpUrl = new URL(params[0]);
HttpURLConnection conn = (HttpURLConnection)phpUrl.openConnection();
if ( conn != null ) {
conn.setConnectTimeout(10000);
conn.setUseCaches(false);
if ( conn.getResponseCode() == HttpURLConnection.HTTP_OK ) {
BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream(), "UTF-8"));
while ( true ) {
String line = br.readLine();
if ( line == null )
break;
jsonHtml.append(line + "\n");
}
br.close();
}
conn.disconnect();
}
} catch ( Exception e ) {
e.printStackTrace();
}
return jsonHtml.toString();
}
protected void onPostExecute(String str) {
try {
// PHP에서 받아온 JSON 데이터를 JSON오브젝트로 변환
JSONObject jObject = new JSONObject(str);
// results라는 key는 JSON배열로 되어있다.
JSONArray results = jObject.getJSONArray("results");
String zz = "";
zz += "Status : " + jObject.get("status");
zz += "\n";
zz += "Number of results : " + jObject.get("num_result");
zz += "\n";
zz += "Results : \n";
for ( int i = 0; i < results.length(); ++i ) {
JSONObject temp = results.getJSONObject(i);
zz += "\tdoc_idx : " + temp.get("id");
zz += "\tmember_idx : " + temp.get("password");
zz += "\tsubject : " + temp.get("name");
zz += "\tcontent : " + temp.get("role");
zz += "\n\t--------------------------------------------\n";
}
tv.setText(zz);
} catch (JSONException e) {
e.printStackTrace();
}
}
}
}
이것은 안드로이드 코드이고요
<head>
<meta http-equiv = "content-Type" content = "text/html" charset = "utf-8">
</head>
<?
$connect = mysqli_connect("127.0.0.1:3306","test","test","test_database");
$sql = "SELECT * FROM nuseInfo";
$result=mysqli_query($connect, $sql);
while($e=mysqli_fetch_assoc($result))
$output[]=$e;
print (json_encode($output));
?>
위는 php 코드입니다.

위 사진은 php를 서버에서 그냥 돌렸을 때의 json 값이고요
에러 logcat은 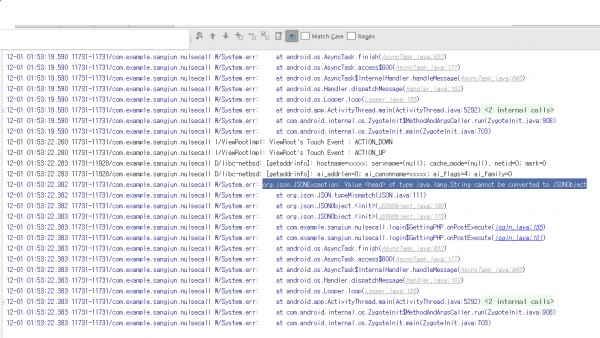
위와 같이 logcat은 구성되네요ㅠㅠㅠㅠ이게 지금 드래그 한부분 저쪽 부분이 문제인 것같은데
찾아보니 다들 들어올 때 방식이 잘못되어 읽지를 못한다고 그러네요ㅠㅠ그럼이거 어케해야되는지ㅠㅠㅠ
해결법좀 가르쳐 주세요
부탁좀드리겠습니다하.ㅠㅠㅠㅠㅠ
log cat이 안보일수 있어서 복붙도 합니다!
hostname=xxxxx; servname=(null); cache_mode=(null), netid=0; mark=0
12-01 01:53:22.283 11731-11928/com.example.sangjun.nulsecall D/libc-netbsd: [getaddrinfo]: ai_addrlen=0; ai_canonname=xxxxx; ai_flags=4; ai_family=0
12-01 01:53:22.382 11731-11731/com.example.sangjun.nulsecall W/System.err: org.json.JSONException: Value <head> of type java.lang.String cannot be converted to JSONObject
12-01 01:53:22.382 11731-11731/com.example.sangjun.nulsecall W/System.err: at org.json.JSON.typeMismatch(JSON.java:111)
12-01 01:53:22.382 11731-11731/com.example.sangjun.nulsecall W/System.err: at org.json.JSONObject.<init>(JSONObject.java:160)
12-01 01:53:22.383 11731-11731/com.example.sangjun.nulsecall W/System.err: at org.json.JSONObject.<init>(JSONObject.java:173)