Paging Library를 이용해서 데이터를 불러오는데 그냥 RecyclerView 에서는 잘 되는데 NestedScrollView나 RecyclerView 안에서 사용하면 모든 페이지가 불러와 지더라구요. 그리고 아이템 재활용도 안되는 것 같습니다.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/section_list"
android:layout_width="match_parent"
android:nestedScrollingEnabled="false"
android:layout_height="wrap_content" />
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical"
android:layout_height="wrap_content">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/content_title"
fontPath="@string/NanumBarunGothicBold_path"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="12.3dp"
android:layout_marginTop="20dp"
android:layout_marginBottom="20dp"
android:includeFontPadding="false"
android:text="@string/text_applied_company"
android:textColor="@color/white"
android:textSize="30sp" />
<TextView
android:id="@+id/manage_btn"
fontPath="@string/NanumBarunGothicUltraLight_path"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@id/content_title"
android:layout_alignParentEnd="true"
android:layout_marginEnd="14dp"
android:foreground="?attr/selectableItemBackground"
android:includeFontPadding="false"
android:text="@string/text_manage"
android:textColor="@color/white"
android:textSize="12sp"
android:visibility="gone" />
</RelativeLayout>
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/content_list"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="@dimen/margin_content_list"
android:layout_marginTop="12dp"
android:layout_marginEnd="@dimen/margin_content_list"
android:layout_marginBottom="10dp" />
<FrameLayout
android:id="@+id/empty_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:visibility="gone">
<com.airbnb.lottie.LottieAnimationView
android:id="@+id/empty_anim"
android:layout_width="300dp"
android:layout_height="300dp"
android:layout_gravity="center"
app:lottie_fileName="lottie/not_found.json"
app:lottie_loop="true"
app:lottie_scale="0.2" />
<TextView
fontPath="@string/NanumBarunGothicBold_path"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="30dp"
android:text="@string/empty_text" />
</FrameLayout>
</FrameLayout>
</LinearLayout>
프래그먼트 부분 XML과 아이템 XML(최상단 RecyclerView)
fun bind(section: Section) {
content_title.text = section.title
if (section.viewType == VIEW_MOVIE) {
manage_btn.visibility = View.GONE
val gridLayoutManager = GridLayoutManager(context, 2)
content_list.layoutManager = gridLayoutManager
content_list.addItemDecoration(MovieItemDecoration(context as Activity?))
val config = PagedList.Config.Builder()
.setInitialLoadSizeHint(PAGE_SIZE)
.setPageSize(PAGE_SIZE)
.build()
val factory = object : DataSource.Factory<Int, Movie>() {
override fun create(): DataSource<Int, Movie> {
val movieApi = MovieApi.create()
return TMMPageKeyDataSource(movieApi = movieApi, region = Util.getRegionCode(context))
}
}
val builder = RxPagedListBuilder(factory, config)
movieAdapter = MoviePagedListAdapter({ view, position ->
val intent = Intent(context, DetailActivity::class.java)
intent.putExtra("movie_id", movieAdapter.getItem(position)?.id)
val poster = Pair.create(view.findViewById<View>(R.id.movie_list_recyclerview_poster), ViewCompat.getTransitionName(view.findViewById(R.id.movie_list_recyclerview_poster)))
val options = ActivityOptionsCompat.makeSceneTransitionAnimation((view.context as Activity), poster)
context.startActivity(intent, options.toBundle())
}, object : OnEmptyListener {
override fun onEmpty() {
setMovieRecyclerEmpty(true)
}
override fun onNotEmpty() {
setMovieRecyclerEmpty(false)
}
})
content_list.adapter = movieAdapter
builder.buildObservable()
.subscribe {
movieAdapter.submitList(it)
}
}
if (section.viewType == VIEW_COMPANY) {
manage_btn.visibility = View.VISIBLE
manage_btn.setOnClickListener(View.OnClickListener { v: View? -> context.startActivity(Intent(context, FavoriteListActivity::class.java)) })
val linearLayoutManager = LinearLayoutManager(context)
linearLayoutManager.orientation = RecyclerView.HORIZONTAL
content_list.layoutManager = linearLayoutManager
companyAdapter = FragmentCompanyFavoriteRecyclerViewAdapter(FavoriteCompanyDataLocalSource.getInstance().getFavoriteCompany(context), { view, position ->
val intent = Intent(view.context, MovieListActivity::class.java)
intent.putExtra("keyword", companyAdapter.getItem(position))
view.context.startActivity(intent)
}, object : OnEmptyListener {
override fun onEmpty() {
setCompanyRecyclerEmpty(true)
}
override fun onNotEmpty() {
setCompanyRecyclerEmpty(false)
}
})
content_list.adapter = companyAdapter
}
}
최상단 RecyclerView의 뷰홀더에서 바인딩 시켜주는 부분
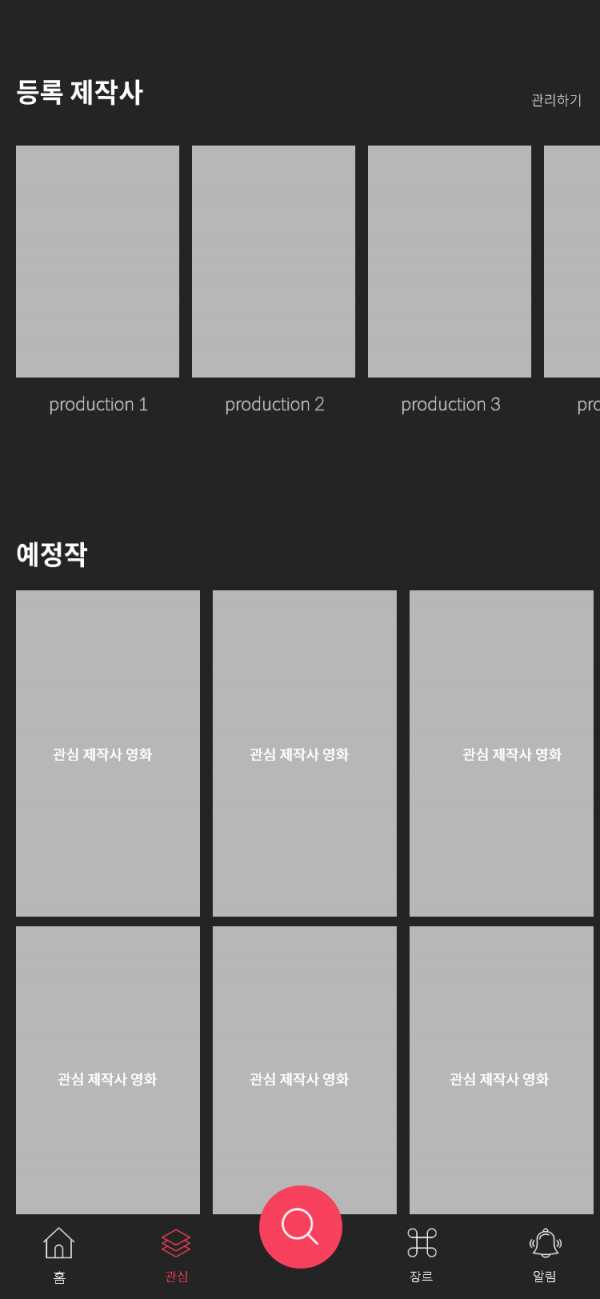
구현하고자 하는 화면이고 위에있는 Horizental 리스트는 로컬에서 불러오는 거고 아래 예정작 리스트가 페이징 하려고 합니다.