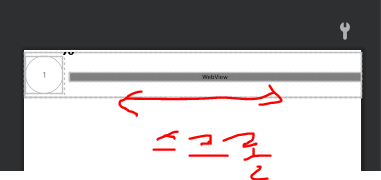
Xml Source
<com.nsdevil.ubttabletv4.views.MathView
android:id="@+id/math_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="10dp"
android:layout_marginTop="10dp"
app:math_json="@{answer.answer}"
app:layout_constraintBottom_toBottomOf="@id/b_answer"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="@id/v_guide_1"
app:layout_constraintTop_toTopOf="parent" />
Kt Source
class MathView : WebView {
private var text: String? = null
@Volatile
private var pageLoaded = false
constructor(context: Context) : super(context) {
init(context)
}
constructor(context: Context, attrs: AttributeSet?) : super(context, attrs) {
init(context)
}
@SuppressLint("SetJavaScriptEnabled")
private fun init(context: Context) {
setBackgroundColor(Color.TRANSPARENT)
text = ""
pageLoaded = false
// enable javascript
settings.apply {
loadWithOverviewMode = true
javaScriptEnabled = true
defaultFontSize = 25
cacheMode = WebSettings.LOAD_CACHE_ELSE_NETWORK
}
// caching
val dir = context.cacheDir
if (!dir.exists()) {
Log.d(TAG, "directory does not exist")
val mkdirsStatus = dir.mkdirs()
if (!mkdirsStatus) {
Log.e(TAG, "directory creation failed")
}
}
// disable click
isClickable = false
isLongClickable = false
settings.useWideViewPort = true
loadUrl("file:///android_asset/www/MathLive.html")
webViewClient = object : WebViewClient() {
override fun onPageFinished(view: WebView, url: String) {
pageLoaded = true
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP_MR1) {
evaluateJavascript("showFormula('$text')", null)
} else {
loadUrl("javascript:showFormula('$text')")
}
super.onPageFinished(view, url)
}
}
}
fun setText(text: String?) {
this.text = text
if (pageLoaded) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP_MR1) {
evaluateJavascript("showFormula('" + this@MathView.text + "')", null)
} else {
loadUrl("javascript:showFormula('" + this@MathView.text + "')")
}
} else {
Log.e(TAG, "Page is not loaded yet.")
}
}
companion object {
private val TAG = MathView::class.java.simpleName
}
}