메모장을 만들고 있습니다
그런데 어플을 실행할때마다 자꾸 null값으로된 리사이클러뷰가 생성되는데 어떻게 해결할지 몰라서 질문합니다
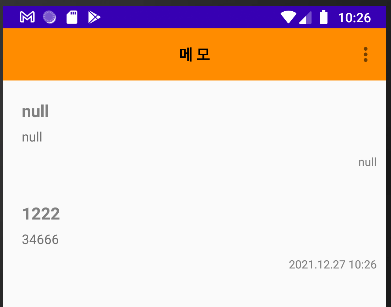
class SqliteHelper(context: Context, name: String, version: Int):
SQLiteOpenHelper(context, name, null, version) {
override fun onCreate(db: SQLiteDatabase?) {
val create = "create table memo (" +
"no integer primary key," +
"caption text," +
"content text," +
"datetime text" +
")"
db?.execSQL(create)
}
override fun onUpgrade(db: SQLiteDatabase?, oldVersion: Int, newVersion: Int) {}
fun insertMemo(memo: Memo) {
val values = ContentValues()
values.put("caption", memo.caption)
values.put("content", memo.content)
values.put("datetime", memo.datetime)
val wd = writableDatabase
wd.insert("memo", null, values)
wd.close()
}
@SuppressLint("Range")
fun selectMemo(): MutableList<Memo> {
val list = mutableListOf<Memo>()
val select = "select * from memo"
val rd = readableDatabase
val cursor = rd.rawQuery(select, null)
while (cursor.moveToNext()) {
val no = cursor.getLong(cursor.getColumnIndex("no"))
val caption = cursor.getString(cursor.getColumnIndex("caption"))
val content = cursor.getString(cursor.getColumnIndex("content"))
val datetime = cursor.getString(cursor.getColumnIndex("datetime"))
list.add(Memo(no, caption, content, datetime))
}
cursor.close()
rd.close()
return list
}
fun updateMemo(memo: Memo) {
val values = ContentValues()
values.put("caption", memo.caption)
values.put("content", memo.content)
values.put("datetime", memo.datetime)
val wd = writableDatabase
wd.update("memo", values, "no = ${memo.no}", null)
}
fun deleteMemo(memo: Memo) {
val delete = "delete from memo where no = ${memo.no}"
val db = writableDatabase
db.execSQL(delete)
db.close()
}
}
data class Memo(var no: Long?, var caption: String, var content: String, var datetime: String)
첫 코드는 sqlite 클래스입니다..
class MainActivity : AppCompatActivity() {
private val binding by lazy { ActivityMainBinding.inflate(layoutInflater) }
val helper = SqliteHelper(this, "memo", 1)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(binding.root)
val adapter = MemoAdapter()
adapter.listData.addAll(helper.selectMemo())
binding.recyclerView.adapter = adapter
binding.recyclerView.layoutManager = LinearLayoutManager(this)
binding.btnCreateMemo.setOnClickListener {
val intent = Intent(this, CreateMeMoActivity::class.java)
startActivity(intent)
}
val title = intent.getStringExtra("title").toString()
val content = intent.getStringExtra("content").toString()
val time = intent.getStringExtra("date").toString()
Log.d(">>", "getString title = ${title}")
Log.d(">>", "getString content = ${content}")
Log.d(">>", "getString date = ${time}")
val memo = Memo(null, title, content, time)
helper.insertMemo(memo)
adapter.listData.clear()
adapter.listData.addAll(helper.selectMemo())
adapter.notifyDataSetChanged()
}
}
두번째 코드는 다른 액티비티에서 인텐트로 값을 전달받은 후 toString() 하여 리사이클러뷰를 생성하는 코드입니다..
어떻게 하면 해결할 수 있는지 알려주시면 감사하겠습니다...